OneSpan Sign How To: Télécharger des documents dans une plage de dates
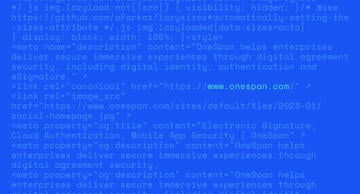
Lorsque vous remplissez un package de documents (transaction dans la nouvelle interface interface, vous avez la possibilité de télécharger vos documents signés. Actuellement, OneSpan Sign conservera tous les paquets remplis indéfiniment. Ainsi, vous pouvez télécharger vos documents signés quand vous le souhaitez. Toutefois, vous pouvez également conserver une copie des documents signés à votre fin. Par exemple, la politique de l'entreprise peut vous obliger à imprimer des copies papier des documents signés, ou vous pouvez simplement les conserver sur vos propres bases de données. La façon la plus simple est de passer par le portail Web OneSpan Sign et télécharger vos documents signés là-bas. Bien que, ce processus peut être long et fastidieux si vous avez un grand nombre de paquets dans votre compte. Dans ce blog, je vais vous montrer comment tirer parti de la fonctionnalité de téléchargement afin de télécharger vos documents signés dans une plage de date.
Le code
Vous pouvez aller de l'avant et passer à la section qui s'applique à votre méthode. Je vais couvrir les mêmes informations dans chaque segment. Vous pouvez obtenir le code d'exemple complet à partir de la part de code communautaire développeur: Java, .NET, et REST.
Java SDK
Je vais commencer par le Java SDK. La première étape consiste à définir votre plage de dates que vous souhaitez récupérer vos documents.
Calendrier cal - nouveau Calendrier grégorien(); cal.add (Calendar.DAY_OF_MONTH, -7); Date de cal.getTime(); Date à la nouvelle date();
Ensuite, vous récupérez vos paquets dans votre plage de dates avec OneSpan Sign's PackageService(), qui vient sous la forme d'une page de OneSpan Sign. Dans l'exemple de code ci-dessous, le nombre de paquets retournés dans la PageRequest est réglé à 50, soit le nombre maximum de paquets que vous pouvez récupérer par page. La première valeur de la PageRequest (indice variable) est le point de départ de la liste globale des paquets qui doivent être retournés. Cette variable est incrémentée que vous passez à travers vos paquets pour garder une trace de l'endroit où nous sommes dans la liste globale des paquets.
Page<documentpackage> completed_packages 'client.getPackageService().getUpdatedPackagesWithinDateRange(PackageStatus.COMPLETED, new PageRequest(index, 50), from, to);</documentpackage>
Ensuite, vous téléchargez votre fichier zip à partir de chacun des paquets complétés dans la page retournée par OneSpan Sign. Une fois que tous les fichiers zip ont été téléchargés, vous mettez à jour votre page pour récupérer les 50 paquets suivants jusqu'à ce que tous les paquets aient été récupérés dans votre plage de date.
tandis que (index= completed_packages.getNumberOfElements())> { pour (DocumentPackage pack : completed_packages) { System.out.println ("Télécharger le paquet: " - pack.getId() -"); octte[] zipContent - client.downloadZippedDocuments(pack.getId()); Files.saveTo(zipContent, "package-documents-" pack.getId().toString() -".zip"); l'indexMD; Thread.sleep (1000); } completed_packages - client.getPackageService().getUpdatedPackagesWithinDateRange(PackageStatus.COMPLETED, new PageRequest(index, 50), from, to); }
SDK .NET
Ensuite, passons en plus de la SDK .NET. La première étape consiste à récupérer vos paquets dans votre plage de dates avec OneSpan Sign's PackageService(), qui vient comme une page de OneSpan Sign. Dans l'exemple de code ci-dessous, le nombre de paquets retournés dans la PageRequest est réglé à 50, soit le nombre maximum de paquets que vous pouvez récupérer par page. La première valeur de la PageRequest (indice variable) est le point de départ de la liste globale des paquets qui doivent être retournés. Cette variable est incrémentée que vous passez à travers vos paquets pour garder une trace de l'endroit où nous sommes dans la liste globale des paquets.
Page<documentpackage> completed_packages - eslClient.PackageService.GetUpdatedPackagesWithinDateRange(DocumentPackageStatus.COMPLETED, new PageRequest(index, 50), DateTime.Today.AddDays(-7), DateTime.Now);</documentpackage>
Ensuite, vous téléchargez votre fichier zip à partir de chacun des paquets complétés dans la page retournée par OneSpan Sign. Une fois que tous les fichiers zip ont été téléchargés, vous mettez à jour votre page pour récupérer les 50 paquets suivants jusqu'à ce que tous les paquets aient été récupérés dans votre plage de date.
tandis que (index= completed_packages.TotalElements)> { foreach (DocumentPackage package en completed_packages) { Debug.WriteLine("Télécharger le paquet: " paquet. Id "..."); octte[] zipContent - eslClient.DownloadZippedDocuments(package. Id); File.WriteAllBytes (Directory.GetCurrentDirectory() -/paquet-documents-"paquet. Id.ToString() ".zip", zipContent); l'indexMD; Thread.Sleep (1000); } completed_packages - eslClient.packageService.GetUpdatedPackagesWithinDateRange(DocumentPackageStatus.COMPLETED, new PageRequest(index, 50), DateTime.Today.AddDays(-7), DateTime.Now); }
API REST
Enfin, je vais passer en charge l'API REST. La première étape consiste à récupérer vos colis dans votre plage de date en faisant une demande GET pour
https://sandbox.esignlive.com/api/packages?query=inbox&lastUpdatedStartDate=2016-06-16&lastUpdatedEndDate=2016-06-23&predefined=completed
Vous devrez définir votre paramètre de plage de date dans l'URL ci-dessus. Ensuite, vous téléchargez votre fichier zip à partir de chacun des paquets remplis dans l'objet JSON retourné par OneSpan Sign.
foreach (paquet var en paquets) { Debug.WriteLine ("Télécharger le paquet: " 'paquet["id"] '..."); var downloadZipResponse - myClient.GetAsync (nouveau Uri (apiUrl -/packages/" ' paquet["id"] -"/documents/zip")). Résultat; Contenu ByteArrayContent - nouveau ByteArrayContent(downloadZipResponse.Content.ReadAsByteArrayAsync(). Résultat); File.WriteAllBytes("C:/Users/hhaidary/Desktop/package-documents-" paquet["id"] - ".zip", contenu. ReadAsByteArrayAsync(). Résultat); Thread.Sleep (1000); }
Exécution de votre code
Vous pouvez maintenant aller de l'avant et exécuter votre code. Si vous avez suivi ma convention de nommage pour les fichiers zip, ci-dessous est une capture d'écran de la sortie de la console, vous pouvez vous attendre une fois que vous avez exécuté votre code.
Si vous avez des questions concernant ce blog ou toute autre chose concernant l'intégration oneSpan Sign dans votre application, visitez les forums de la communauté des développeurs: https://developer.OneSpan.com. C'est moi qui l'ai fait. Merci de lire! Si vous avez trouvé ce message utile, s'il vous plaît le partager sur Facebook, Twitter, ou LinkedIn.
Haris Haidary
Évangéliste technique junior
LinkedIn - France Twitter (en)