OneSpan Sign How To: Demander des pièces jointes
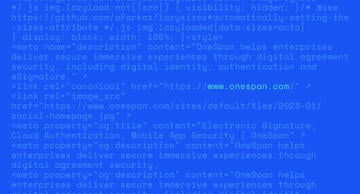
En tant qu'expéditeur, vous pouvez demander à un signataire de télécharger une pièce jointe pendant la cérémonie de signature. Par exemple, en tant que courtier d'assurance automobile, vous pouvez exiger de votre client qu'il fournisse une copie de son permis de conduire ainsi que les documents signés. Une fois les pièces jointes demandées fournies, vous aurez la possibilité d'accepter ou de rejeter la pièce jointe. En cas de refus, vous serez en mesure de fournir des commentaires sous la forme d'un court message et le signataire aura la possibilité de télécharger un fichier différent. Dans ce blog, je vais vous montrer comment demander, télécharger et accepter / refuser des pièces jointes de fichiers avec le OneSpan Sign Java SDK, .NET SDK, et REST API.
Le code
Maintenant que nous connaissons notre objectif, nous allons plonger directement dans le code. Je vais couvrir exactement les mêmes informations dans chaque segment. Allez-y et passez à la section qui s'applique à vous. Code d'exemple complet pour ce blog peut être trouvé dans le développeur Community Code Share: Java, .NET, et REST.
Java SDK
Je vais commencer par le Java SDK. Vous trouverez ci-dessous un exemple de code sur la façon dont vous modifieriez votre bloc de signataires pour demander une pièce jointe au fichier. La méthode withDescription()
vous permet de fournir une description au signataire sur le téléchargement de fichiers que vous recherchez et la méthode isRequiredAttachment()
définit que vous avez besoin de la pièce jointe. Ni l'un ni l'autre ne sont obligatoires lors de la construction de votre objet AttachmentRequirement.
Si vous avez besoin d'une comparaison avec la création d'objet de document de base ou si c'est la première fois que vous créez un package avec le Java SDK, consultez ce blog.
.withSigner(newSignerWithEmail("[email protected]") .avecFirstName ("John") .avecLastName ("Doe") .withCustomId("Signer1") .withAttachmentRequirement(newAttachmentRequirementWithName("Driver's license") .withDescription ("S'il vous plaît télécharger une copie de votre permis de conduire.") .isRequiredAttachment() .construire()))
Par conséquent, vous pouvez également interroger l'état de chaque pièce jointe de votre paquet. Le signe OneSpan renvoie les objets AttachmentRequirement
sous forme de liste. Le code ci-dessous bouclera chaque objet AttachmentRequirement
et imprimera le nom, l'état et l'id de chaque pièce jointe demandée pour ce signataire particulier.
DocumentPackage myPackage - client.getPackage (packageId); Liste<attachmentrequirement> signer1Attachments - myPackage.getSigner("[email protected]"). getAttachmentRequirements();</attachmentrequirement> pour (AttachmentRequirement attachment : sign1Attachments) System.out.println (attachment.getName() - " attachment.getStatus() - " - attachment.getStatus() " - attachment.getId()); }
En outre, vous avez la possibilité de télécharger des pièces jointes de trois manières différentes:
- Une seule pièce jointe (nécessite l'iD d'attachement)
- Toutes les pièces jointes dans un paquet
- Toutes les pièces jointes d'un signataire particulier
Télécharger la pièce jointe individuelle DownloadedFile downloadAttachment -client.getAttachmentRequirementService().downloadAttachmentFile(packageId, attachmentId); Files.saveTo(downloadedAttachment.getContents(), downloadAttachment.getFilename()); Télécharger toutes les pièces jointes dans le package DownloadedFile downloadedAllAttachmentsForPackage - client.getAttachmentRequirementService().downloadAllAttachmentFilesForPackage(packageId); Files.saveTo(downloadedAllAttachmentsForPackage.getContents(), "downloadedAllAttachmentsForPackage.zip"); Télécharger toutes les pièces jointes pour le signataire dans le paquet DownloadedFile downloadedAllAttachmentsForSigner1InPackage - client.getAttachmentRequirementService().downloadAllAttachmentFilesForSignerInPackage(myPackage, sign1); Files.saveTo(downloadedAllAttachmentsForSigner1InPackage.getContents(), "downloadedAllAttachmentsForSigner.zip");
Après avoir examiné la pièce jointe, vous avez la possibilité de refuser ou d'accepter l'attachement. Dans cette dernière situation, vous pouvez inclure des commentaires sous la forme d'un petit message expliquant le refus.
client.getAttachmentRequirementService().rejectAttachment(packageId, signataire1, "Driver's license", "Expired driver's license");
Il est important de noter que les paquets avec les pièces jointes requises ne seront pas automatiques complets. Il s'agit pour l'expéditeur d'avoir la possibilité d'examiner la pièce jointe et de l'accepter/rejeter. Si toutes les pièces jointes requises ont été téléchargées et approuvées, vous pouvez compléter le package en mettant à jour l'état de votre colis à COMPLETED.
DocumentPackage myPackage - client.getPackage (packageId); myPackage.setStatus (PackageStatus.COMPLETED); client.updatePackage(packageId, myPackage);
SDK .NET
Ensuite, je vais couvrir le .NET SDK. Vous trouverez ci-dessous un exemple de code sur la façon dont vous modifieriez votre bloc de signataires pour demander une pièce jointe au fichier. La méthode WithDescription()
vous permet de fournir une description au signataire sur le téléchargement de fichiers que vous recherchez et la méthode IsRequiredAttachment()
définit que vous avez besoin de la pièce jointe. Ni l'un ni l'autre ne sont obligatoires lors de la construction de votre objet AttachmentRequirement.
Si vous avez besoin d'une comparaison avec la création d'objet document de base ou si c'est la première fois que vous créez un package avec le .NET SDK, voir ce blog.
. WithSigner(SignerBuilder.NewSignerWithEmail("[email protected]") . WithFirstName (" John ") . WithLastName ("Doe") . WithCustomId ("Signer1") . WithAttachmentRequirement(AttachmentRequirementBuilder.NewAttachmentRequirementWithName("Driver's license") . WithDescription ("S'il vous plaît télécharger une copie de votre permis de conduire.") . IsrequiredAttachment() . Construire()))
Par conséquent, vous pouvez également interroger l'état de chaque pièce jointe de votre paquet. Le signe OneSpan renvoie les objets AttachmentRequirement
sous forme de liste. Le code ci-dessous bouclera chaque objet AttachmentRequirement
et imprimera le nom, l'état et l'id de chaque pièce jointe demandée pour ce signataire particulier.
DocumentPackage myPackage - client. GetPackage (packageId); IList<attachmentrequirement> sign1Attachments - myPackage.GetSigner ("[email protected]"). Pièces jointes;</attachmentrequirement> foreach (AttachmentRequirement attachment in sign1Attachments) { Debug.WriteLine (attachement. Nom '" ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' Statut " " - pièce jointe. Id); }
En outre, vous pouvez télécharger des pièces jointes de trois façons différentes :
- Une seule pièce jointe (nécessite l'iD d'attachement)
- Toutes les pièces jointes dans un paquet
- Toutes les pièces jointes d'un signataire particulier
Télécharger la pièce jointe individuelle DownloadedFile downloadedAttachment - client. AttachmentRequirementService.DownloadAttachmentFile(packageId, attachmentId); System.IO.File.WriteAllBytes(downloadedAttachment.Filename, downloadedAttachment.Contents); Télécharger toutes les pièces jointes dans le package DownloadedFile downloadedAllAttachmentsForPackage - client. AttachmentRequirementService.DownloadAllAttachmentFilesForPackage(packageId); System.IO.File.WriteAllBytes("downloadedAllAttachmentsForPackage.zip", downloadedAllAttachmentsForPackage.Contents); Télécharger toutes les pièces jointes pour le signataire dans le paquet DownloadedFile downloadedAllAttachmentsForSigner1InPackage - client. AttachmentRequirementService.DownloadAllattachmentFilesForSignerInPackage (myPackage, signataire1); System.IO.File.WriteAllBytes("downloadedAllAttachmentsForSigner.zip", downloadedAllAttachmentsForSigner1InPackage.Contents);
Après avoir examiné la pièce jointe, vous avez la possibilité de refuser la pièce jointe. Dans cette situation, vous pouvez inclure des commentaires sous la forme d'un petit message expliquant le refus.
Client. AttachmentRequirementService.RejectAttachment(packageId, sign1, "Driver's license", "Expired driver's license");
Il est important de noter que les paquets avec les pièces jointes requises ne seront pas automatiques complets. Il s'agit pour l'expéditeur d'avoir la possibilité d'examiner la pièce jointe et de l'accepter/rejeter. Si toutes les pièces jointes requises ont été téléchargées et approuvées, vous pouvez compléter le package en mettant à jour l'état de votre colis à COMPLETED.
DocumentPackage myPackage - eslClient.GetPackage(packageId); myPackage.Status - DocumentPackageStatus.COMPLETED; eslClient.UpdatePackage(packageId, myPackage);
API REST
Enfin, je couvrirai l'API REST. L'exemple JSON ci-dessous montre comment modifier vos rôles objet de demander une pièce jointe. Si vous avez besoin d'une comparaison avec la création d'objets de document de base ou si c'est la première fois que vous créez un package avec l'API REST, consultez ce blog.
[code language"javascript"] "rôles": [ - "id": "client", "type": "SIGNER", "index": 1, "attachmentRequirements": [ - "description": "S'il vous plaît télécharger une copie numérisée de votre permis de conduire.", "requis": vrai, "id": "lD6p5QnWk905", "données": null, "status": "INCOMPLETE", "commentaire": "", "nom": "Driver's@gmaillicense" "signataires": [ - "premier nom": "John", "lastName": "Smith", "email": "john.smith .com" - ], "nom": "client" , "id": "entrepreneur", "type": "SIGNER", "index": 2, "signataires": [ email": "[email protected]" - ], "nom": "entrepreneur" - ] En conséquence, vous pouvez également demander l'état de chaque pièce jointe dans votre paquet. Le code ci-dessous récupérera chaque objet de fixationDans votre paquet.
HttpClient myClient ' nouveau httpClient(); myClient.DefaultRequestHeaders.Authorization - new AuthenticationHeaderValue ("Basic", apiKey); myClient.DefaultRequestHeaders.Add("Accept", "application/json"); var rôles - myClient.GetAsync (nouveau Uri (apiUrl - "/packages/ 'packageId/roles").). Résultat; JObject roles_json = JObject.Parse(roles. Content.ReadAsStringAsync(). Résultat); résultats var - roles_json["résultats"]; foreach (attachement var dans les résultats) { var attacher ' attachement["attachmentRequirements"]; Debug.WriteLine (attacher); }
Pour télécharger une pièce jointe individuelle, vous aurez besoin du paquet et de l'id de pièce jointe. L'exemple de code ci-dessous vous montre comment faire votre demande :
var attachment ' myClient.GetAsync(nouveau Uri(API_URL '//packages/'packageId/attachment/'attachmentId').). Résultat; Contenu ByteArrayContent et nouveau ByteArrayContent(pièce jointe). Content.ReadAsByteArrayAsync(). Résultat); Fichier.WriteAllBytes ("PATH_TO_DOWNLOAD", contenu. ReadAsByteArrayAsync(). Résultat);
Pour télécharger toutes les pièces jointes comme un fichier zip dans un paquet, vous ferez votre demande à https://sandbox.onespan.com/api/packages/ 'packageId'/attachment/zip
. Pour télécharger toutes les pièces jointes comme un fichier zip pour un signataire particulier, vous ferez votre demande à https://sandbox.onespan.com/api/packages/ 'packageId'/attachment/zip/'roleId.
Après avoir examiné la pièce jointe, vous avez la possibilité d'accepter ou de refuser la pièce jointe. Dans cette dernière situation, vous pouvez inclure des commentaires sous la forme d'un petit message expliquant le refus. Pour refuser une pièce jointe, vous demanderez à https://sandbox.onespan.com/api/packages/'packageId/roles/'role/'roleId'
avec le JSON suivant : [code language'"javascript"]' "attachmentRequirements": [ ' "id": "q66CYiDrxTU1", "status": "REJECTED", "commentaire": "Invalid copy." Il est important de noter que les paquets avec les pièces jointes requises ne seront pas automatiques complets. Il s'agit pour l'expéditeur d'avoir la possibilité d'examiner la pièce jointe et de l'accepter/rejeter. Si toutes les pièces jointes requises ont été téléchargées et approuvées, vous pouvez compléter le package en faisant une demande PUT à https://sandbox.onespan.com/api/packages/'packageId,
avec le JSON suivant: [code language'"javascript"] "status" : "COMPLETED"
Exécution de votre code
Une fois que vous avez exécuté votre code, si vous vous connectez à OneSpan Sign et que vous vous dirigez vers les paramètres du signataire dans votre paquet, vous devez trouver vos demandes de pièces jointes dans l'onglet pièces jointes.
Pendant la cérémonie de signature, le signataire sera présenté au dialogue ci-dessous demandant de télécharger la pièce jointe requise.
Si vous naviguez vers votre répertoire d'espace de travail, vous trouverez vos pièces jointes téléchargées.
Et voilà. Vous avez demandé avec succès des pièces jointes! Si vous avez des questions concernant ce blog ou toute autre chose concernant l'intégration de OneSpan Sign dans votre application, visitez les forums de la communauté des développeurs: https://developer.onespan.com.
C'est moi qui l'ai fait. Merci de lire! Si vous avez trouvé ce message utile, s'il vous plaît le partager sur Facebook, Twitter, ou LinkedIn.
Haris Haidary
Évangéliste technique junior
LinkedIn - France Twitter (en)