OneSpan Sign How To: Création d'une application Web PHP simple
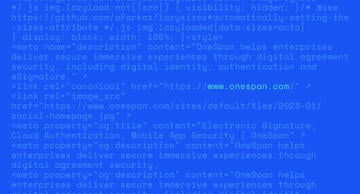
PHP est un langage de script côté serveur conçu principalement pour le développement web. C'est l'un des langages de script les plus prédominants utilisés par les développeurs web. Les principales raisons de sa popularité sont les suivantes:
- Facile à apprendre
- Bien documenté
- Facile à utiliser
PHP a de loin la plus petite courbe d'apprentissage. Tout ce que vous devez commencer est quelques balises PHP à l'intérieur d'un fichier HTML et voir le résultat. Ensuite, PHP est bien documenté. Vous pouvez trouver des tonnes d'exemples sur le site officiel de PHP ou simplement en faisant une recherche en ligne. Cela est dû au vaste soutien communautaire de PHP. Enfin, il est particulièrement facile à utiliser. Contrairement à la plupart des solutions telles que Java et C, le code PHP n'a pas besoin d'être compilé. Vous pouvez simplement écrire votre script et rafraîchir votre navigateur pour voir les résultats. Dans ce blog, je vais vous montrer comment créer une application web PHP simple dans laquelle votre utilisateur est tenu de remplir un formulaire et ensuite signer un contrat d'échantillon en utilisant OneSpan Sign.
Exigences
Avant de plonger dans le code, vous devrez configurer votre environnement pour créer votre application web PHP. Pour ce blog, tout ce dont vous avez besoin est un serveur web avec un interprète PHP et un éditeur de texte.
Téléchargement XAMPP Je vais utiliser XAMPP, qui est un serveur web open-source gratuit avec des interprètes PHP et PERL. Il est simple, léger et vous permet de tester vos scripts localement. Vous pouvez obtenir XAMPP à partir du lien ci-dessous.
Téléchargement d'un éditeur de texte
Vous aurez également besoin d'un éditeur de texte. Pour ce blog, je vais utiliser Sublime Text. Vous pouvez télécharger une copie gratuite à partir du lien ci-dessous.
Installation et configuration XAMPP
Après avoir téléchargé XAMPP, allez-y et exécutez le fichier exécutable. Si vous avez un programme antivirus en cours d'exécution sur votre ordinateur, vous pourriez être invité si vous voulez continuer avec l'installation. Cliquez sur "Oui". Il est recommandé d'éteindre votre antivirus lors de l'exécution de XAMPP.
Ensuite, vous serez présenté avec la page de bienvenue XAMPP. Cliquez sur "suivant". Ensuite, il vous sera demandé de sélectionner les composants que vous souhaitez installer. Pour les besoins de ce blog, vous aurez seulement besoin du serveur Apache et PHP comme langage de programmation.
Après avoir cliqué sur "Suivant", il vous sera demandé de sélectionner un dossier pour installer XAMPP. Dans mon exemple, j'ai choisi de l'installer dans l'annuaire par défaut (par exemple C: xampp).
De là, vous pouvez continuer à cliquer sur "Suivant" jusqu'à ce que l'installation soit terminée. Enfin, cliquez sur "Finish", qui lancera le panneau de contrôle XAMPP.
Le numéro de port par défaut utilisé par XAMPP est de 80. Dans mon exemple, j'ai changé le numéro de port en "1234". Cette étape est purement facultative. Si vous choisissez de le laisser 80, votre URL sera "localhost:80". Pour modifier le numéro de port, cliquez sur "Config" dans le panneau de contrôle et sélectionnez "Apache (httpd.conf)". Dans le fichier de configuration, modifiez les éléments suivants :
Ecouter 80 ServerNom localhost:80
Remplacez-les par :
Écouter 1234 ServerNom localhost:1234
Enregistrez vos modifications et fermez le fichier de configuration. Vous pouvez maintenant exécuter Apache en cliquant sur "Démarrer" dans le panneau de contrôle.
Dans votre navigateur, entrez "localhost:1234". Si vous voyez l'écran ci-dessous, cela signifie que vous avez réussi à installer XAMPP sur votre ordinateur.
Vous pouvez également tester si XAMPP a installé PHP avec succès avec un test simple. Ouvrez sublime texte et tapez ce qui suit:
Enregistrer ce fichier comme "test.php" dans "C: xampp'htdocs". Ensuite, naviguez vers "localhost:1234/test.php". Vous devriez voir le message "Bonjour Word!" :
Le code
Nous sommes maintenant prêts à plonger dans le code. Créez un nouveau dossier sous la discition "C: xampp-htdocs" et nommez-le "esignlive". La première étape consiste à créer votre formulaire HTML. Dans Sublime Text, créez un nouveau fichier et copiez le code ci-dessous :
Veuillez remplir le formulaire suivant :
Prénom:
Nom de famille :
Adresse e-mail :
Société:
Adresse:
Ville:
État:
Zip:
Pays:
Numéro de téléphone :
Numéro de politique :
Comme vous pouvez le voir sur le code HTML ci-dessus, sur soumettre, le formulaire-données seront envoyés à un fichier nommé "thankyou.php" (sera créé sous peu). Enregistrez votre fichier sous le nom de "index.html" dans votre dossier "OneSpan Sign" que vous venez de créer (par exemple. C: xampp-htdocs-esignlive). Dans votre navigateur, si vous naviguez vers localhost:1234/esignlive/index.html, vous devriez voir ce qui suit :
Si vous remplissez le formulaire et cliquez sur "Soumettre", vous obtiendrez une erreur 404 puisque nous n'avons pas encore créé un fichier "thankyou.php". Allons-y et faisons-le.
Dans Sublime Text, créez un nouveau fichier et copiez ce qui suit :
Merci d'avoir rempli le formulaire! Veuillez signer le document ci-dessous :
Enregistrez ce fichier comme "thankyou.php" dans votre dossier "OneSpan Sign". Dénoncons le code ci-dessus. Les deux premières lignes importent le fichier "ESLPackageCreation.php" (sera créé sous peu) et instantanément une nouvelle classe.
exiger ("ESLPackageCreation.php"); $package - nouvelle ESLPackageCreation();
Les deux prochaines lignes sont où nous passons les données de formulaire comme paramètres aux méthodes de la classe "ESLPackageCreation" pour créer et envoyer un paquet, et ensuite construire un jeton d'authentification de signataire.
Dans le code ci-dessus, chaque méthode crée la charge utile JSON appropriée à partir du tableau en utilisant json_encode(). Cette fonction PHP traduit les données transmises à une chaîne JSON, qui peut ensuite être utilisée pour faire une demande cURL à OneSpan Sign. Aussi, assurez-vous de remplacer le porte-clés api avec votre propre valeur.
Remarque: Si vous êtes sur un environnement Mac ou Linux, veuillez utiliser des slashes avant lorsque vous spécifiez les emplacements de chemin de fichiers.
Exécution de votre projet
Vous êtes maintenant prêt à exécuter votre application web PHP. Dans votre navigateur, entrez localhost:1234/esignlive/index.html. Allez-y et remplissez quelques exemples d'informations et cliquez sur "Soumettre".
Vous devriez être redirigé vers la cérémonie oneSpan Sign. Après avoir accepté le formulaire de consentement par défaut, vous serez présenté avec les champs de contrat d'échantillon peuplés avec les données que vous avez entrés dans le formulaire et le contrat prêt à être signé.
Si vous avez des questions concernant ce blog ou toute autre chose concernant l'intégration oneSpan Sign dans votre application, visitez les forums de la communauté des développeurs: https://developer.esignlive.com.
C'est moi qui l'ai fait. Merci de lire! Si vous avez trouvé ce message utile, s'il vous plaît le partager sur Facebook, Twitter, ou LinkedIn.
Haris Haidary
Évangéliste technique junior
LinkedIn - France Twitter (en)