OneSpan Signe Comment: Créer des groupes
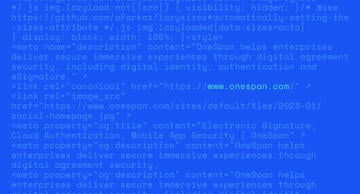
Un groupe est une collection de signataires qui peuvent agir comme un seul signataire du point de vue du créateur du paquet. Cela peut être utile si vous avez besoin d'un membre d'un groupe de votre organisation pour signer un document (c.-à-d. quelqu'un des RH). Vous pouvez y parvenir dans OneSpan Sign en créant un groupe et attribuer la signature au groupe. Dans ce blog, je vais vous montrer comment créer, gérer et utiliser des groupes avec le OneSpan Sign Java SDK, .NET SDK, et REST API.
Groupes dans l'interface uI
La première chose que vous voulez faire est de localiser des groupes dans l'interface du travail. Après vous être déconnecter de votre compte OneSpan Sign, cliquez sur GROUPS dans la barre d'outils. Après avoir exécuter votre code, vous trouverez tous vos groupes ici, comme indiqué dans la section "Running Your Code" ci-dessous . Vous pouvez toujours créer des groupes directement à partir de l'interface du travail si c'est ce que vous souhaitez. Vous n'avez pas besoin d'aller plus loin dans ce blog. Si vous ne voyez pas l'option GROUPS dans la barre d'outils, ils ne sont probablement pas activés sur votre compte. S'il vous plaît contacter notre équipe de soutien à @e-signlivesupport .com. [promotion id'"14704"]
Le code
Vous pouvez aller de l'avant et passer à la section qui s'applique à votre technologie. Je vais couvrir les mêmes informations dans chaque segment. Code d'exemple complet pour ce blog peut être trouvé dans le développeur Community Code Share: Java, .NET, et REST. JAVA SDK (en) Commençons par le Java SDK. Le code d'exemple ci-dessous crée un objet de groupe appelé "Java Developers" avec deux membres. Il est important de noter que ces membres doivent être des expéditeurs dans votre compte. La méthode withIndividualMemberEmailing() enverra des e-mails aux membres du groupe au lieu de l'e-mail de groupe spécifié. Si vous souhaitez envoyer des e-mails au groupe e-mail à la place, vous utiliseriez sans IndividualEmailing(). Ensuite, vous faites appel à votre OneSpan Sign GroupService pour créer votre groupe.
Groupe 1 - GroupBuilder.newGroup ( "Java Developers" ) .withEmail ( "[email protected]" ) .withIndividualMemberEmailing() .withMember( GroupMemberBuilder.newGroupMember(@mycompany"manager .com" ) .as( GroupMemberType.REGULAR ) ) .withMember( GroupMemberBuilder.newGroupMember( "[email protected]" ) .as( GroupMemberType.REGULAR ) ) .construire(); Groupe crééGroup1 - eslClient.getGroupService().createGroup(groupe1);
D'autre part, vous pouvez créer un groupe vide et ensuite inviter les membres à rejoindre votre groupe.
esl.getGroupService().inviteMember( createdEmptyGroup.getId(), GroupMemberBuilder.newGroupMember( "[email protected]" ) .as( GroupMemberType.REGULAR ) .construire() );
La récupération de vos groupes peut être utile lorsque vous souhaitez inviter des membres à votre groupe. Il est important de noter que l'id de groupe est nécessaire lors de l'envoi d'invitations. Vous faites appel à votre OneSpan Sign GroupService pour récupérer vos groupes, qui vous sont retournés sous forme de liste. Dans mon exemple, j'ai récupéré le nom du groupe, l'e-mail de groupe et l'id de groupe pour chaque groupe. Enfin, avec vos ids de groupe, vous pouvez récupérer chaque membre, ainsi que leur e-mail, prénom et nom de famille.
Liste de tous les groupes 'esl.getGroupService').getMyGroups(); pour ( Groupe groupe : allGroups ) System.out.println( group.getName() - avec e-mail " - group.getEmail() et id " - group.getId() ); Liste de tous les membres ' esl.getGroupService().getGroupMembers( group.getId() ); pour ( membre du groupe membre : tous les membres ) System.out.println( member.getGroupMemberType().toString() ' ' ' ' ' ' member.getFirstName() ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' ' } }
Lorsque votre groupe est créé, vous pouvez désormais ajouter un signataire de groupe à votre package. Le code ci-dessous vous montre comment modifier le bloc de signataire afin d'ajouter un signataire de groupe.
DocumentPackage myPackage - newPackageNamed( "Mon paquet avec le groupe Signers Java Developers" ) .withSigner( SignerBuilder.newSignerFromGroup( myGroup.getId() ) .canChangeSigner() .deliverSignedDocumentsByEmail() ) .withDocument( newDocumentWithName( "Mon Document" ) .fromFile ("DOCUMENT_FILE_PATH" ) .withSignature( signaturePour( myGroup.getId() ) .onPage( 0 ) .atPosition( 370, 680 ) ) ) ) ) .construire(); PackageId packageId - esl.createAndSendPackage ( myPackage );
SDK .NET Ensuite, je vais passer en plus de la SDK .NET. Le code de l'échantillon ci-dessous crée un objet de groupe appelé ".NET Developers" avec deux membres. Il est important de noter que ces membres doivent être des expéditeurs dans votre compte. La méthode WithIndividualMemberEmailing() enverra des e-mails aux membres du groupe au lieu de l'e-mail de groupe spécifié. Si vous souhaitez envoyer des e-mails au groupe e-mail à la place, vous utiliseriez WithoutIndividualEmailing(). Ensuite, vous faites appel à votre OneSpan Sign GroupService pour créer votre groupe.
Groupe 1 - GroupBuilder.NewGroup (".NET Developers") . WithMember (GroupMemberBuilder.NewGroupMember("[email protected]") . AsMemberType (GroupMemberType.REGULAR)) . WithMember (GroupMemberBuilder.NewGroupMember("[email protected]") . AsMemberType (GroupMemberType.REGULAR)) . WithEmail ("[email protected]") . WithIndividualMemberEmailing() . Build (); Groupe crééGroup1 - eslClient.GroupService.CreateGroup (groupe1);
D'autre part, vous pouvez créer un groupe vide et ensuite inviter les membres à rejoindre votre groupe.
eslClient.GroupService.InviteMember(crééEmptyGroup.Id, GroupMemberBuilder.NewGroupMember("[email protected]") . AsMemberType (GroupMemberType.REGULAR) . Construire());
La récupération de vos groupes peut être utile lorsque vous souhaitez inviter des membres à votre groupe. Il est important de noter que l'id de groupe est nécessaire lors de l'envoi d'invitations. Vous faites appel à votre OneSpan Sign GroupService pour récupérer vos groupes, qui vous sont retournés sous forme de liste. Dans mon exemple, j'ai récupéré le nom du groupe, l'e-mail de groupe et l'id de groupe pour chaque groupe. Enfin, avec vos ids de groupe, vous pouvez récupérer chaque membre, ainsi que leur e-mail, prénom et nom de famille.
Liste de tous les groupes - eslClient.GroupService.GetMyGroups(); foreach (Groupe de groupe dans tous les groupes) { Debug.WriteLine (groupe. Nom '" avec e-mail " - groupe. Courriel et id , groupe. Id.Id); Liste de tous les membres - eslClient.GroupService.GetGroupMembers(groupe. Id); foreach (membre du groupe membre dans tous les membres) { Debug.WriteLine (membre. GroupMemberType.ToString() '" ' ' membre. FirstName ' " - membre. Dernier nom et " avec e-mail " et membre. Courriel); } }
Lorsque votre groupe est créé, vous pouvez désormais ajouter un signataire de groupe à votre package. Le code ci-dessous vous montre comment modifier le bloc de signataire afin d'ajouter un signataire de groupe.
DocumentPackage superDuperPackage - PackageBuilder.NewPackageNamed("Mon paquet avec les signataires du groupe .NET Developers") . WithSigner(SignerBuilder.newSignerFromGroup(myGroup.Id) . CanChangeSigner() . DeliverSignedDocumentsByEmail()) . WithDocument (DocumentBuilder.NewDocumentNamed(«Mon document») . FromFile ("DOCUMENT_FILE_PATH") . WithSignature(SignatureBuilder.SignatureFor(myGroup.Id) . OnPage(0) . AtPosition (370, 680)))) . Build (); PackageId packageId - eslClient.CreateAndSendPackage(superDuperPackage);
API REST Enfin, je couvrirai l'API REST. La chaîne JSON ci-dessous créera votre groupe avec deux membres en une seule demande. Il est important de noter que dans ce cas, les membres doivent être des expéditeurs dans votre compte. [code language"javascript"] "email": "your_group_email", "nom": "your_group_name", "membres": [ - "en attente": vrai, "email": "[email protected]", "memberType": "REGULAR", "firstName": "John", "lastName": "Doe" , "pending": true, "email": "[email protected]", "memberType": "REGULAR", "firstName": "Mary", "lastName": "Doe" D'autre part, vous pouvez créer un groupe vide et ensuite inviter les membres à rejoindre votre groupe. [code language"javascript"] "email": "[email protected]", "name": "REST Developers" La récupération de vos groupes peut être utile lorsque vous souhaitez inviter des membres à votre groupe. Il est important de noter que l'id de groupe est nécessaire lors de l'envoi d'invitations. Dans ce cas, vous n'avez qu'à faire une demande GET pour https://sandbox.onespan.com/api/groups. Vous pouvez également récupérer un groupe individuel en faisant une demande à https://sandbox.onespan.com/api/groups/groupId. Dans ce cas, vous aurez besoin de l'id du groupe. Voici un exemple de réponse que vous pourriez voir lors de la prise de cette demande pour récupérer vos groupes: [code language'"javascript"] "compte": 1, "résultats": [ - "compte": 'fournisseurs': null, "updated": "2016-01-07T18:49:19Z", "société": "licences": [], "logoUrl": "", "customFields": [], "créé": "2016-01-07T18:49:19Z", "propriétaire": "", "id": "zRcJCHV3ztIB", "données": null, "name": "" , "mise à jour": "2016-01-07T13:58:33Z", "email": "[email protected]", "membres": [ " userId": "1XyqlkXM9uIX", "email": "[email protected]", "firstName": "John", "lastName": "Doe", "memberType": "REGULAR", "pending": false " userId ": "kVooKpofKuUK", "email": "[email protected]", "firstName": "Mary", "lastName": "Doe", "memberType": "REGULAR", "pending": false, "emailMembers": false, "reciprocalDelegation": faux, "créé": "2016-01-07T13:58:33Z", "id": "4a8868d7-1968-4172-aedb-0a9dc8edb683", "data": null, "name": "REST Developers" créé, vous pouvez maintenant ajouter un signataire de groupe à votre paquet. Le code ci-dessous vous montre comment le faire.
apiUrl à cordes et «https://sandbox.onespan.com/api/packages»; string jsonString ' '''role'' : ''cc5d5296-ae92-48ec-a0ee-2a327d8a66b9', ''fields'':[ ''top':680, "gauche":370, "largeur":200, "hauteur":50, "page":0, "type":"SIGNATURE", "valeur":null, "subtype":"FULLNAME" StringContent jsonContent - nouveau StringContent(jsonString, Encoding.UTF8, "application/json"); HttpClient myClient ' nouveau httpClient(); myClient.DefaultRequestHeaders.Authorization - new AuthenticationHeaderValue ("Basic", apiKey); myClient.DefaultRequestHeaders.Add("Accept", "application/json"); var réponse - myClient.PostAsync (nouveau Uri (apiUrl - "//packageId/documents / 'documentId'/approbations"), jsonContent). Résultat; Debug.WriteLine (réponse. Content.ReadAsStringAsync(). Résultat);
Exécution de votre code
Vous pouvez maintenant exécuter votre code. Une fois que vous avez fait cela, vous trouverez vos groupes nouvellement créés dans votre compte OneSpan Sign tel que décrit au début dans la section « Groupes dans l'interface uinterface ». Lors de la récupération de vos groupes avec les SDKs comme indiqué ci-dessus, j'ai inclus le code qui a saisi l'e-mail de groupe et id, et énuméré chaque membre dans la console. Si vous avez parcouru la section API REST, j'ai déjà montré un exemple de réponse de la demande GET déjà. De plus, vous trouverez votre signature de groupe dans votre document comme indiqué ci-dessous. Et voilà. Vous avez créé avec succès des groupes avec OneSpan Sign. Si vous avez des questions concernant ce blog ou toute autre chose concernant l'intégration de OneSpan Sign dans votre application, visitez les forums de la communauté des développeurs: https://developer.onespan.com. C'est moi qui l'ai fait. Merci de lire! Si vous avez trouvé ce message utile, s'il vous plaît le partager sur Facebook, Twitter, ou LinkedIn.
Haris Haidary Junior Technical Product Evangelist LinkedIn (fr) Twitter (en)