OneSpan Sign How To: Delegating Access
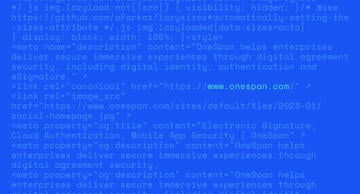
OneSpan Sign gives you the ability to delegate senders access to your account. In other words, you can delegate users to act on your behalf. The delegates will have access to the account holder’s inbox, drafts, layouts, and templates. They will also have the capability of signing documents in your stead. For example, this feature can come in handy when you (e.g. manager) are looking to monitor progress, manage transactions, and retrieve completed documents for employees who are not currently available. It is important to note that all transactions made by the delegates are still owned by the delegator. In this blog, I will show you how to delegate access with the OneSpan Sign web UI, Java SDK, and .NET SDK. Delegating access with the REST API will be available in future releases.
Delegating Access in the UI
To delegate access from the UI, go ahead and login to your OneSpan Sign account. Click on IDENTITY in the toolbar and scroll down to the delegation section. Next, click on ‘delegation’. From there, you have the ability to add and remove delegates from your account. Please note that to add a delegate, the user has to be a sender in your account. After running your code, you will find all your delegates here, as shown in the "Running Your Code" segment below. If delegating access from the UI suffices, you do not need to go any further into this blog. If you are not able to add delegates, they are likely not enabled on your account. Please contact our support team at [email protected] to enable this feature.
The Code
In this segment of the blog, I will cover how to: retrieve all of your senders, add/remove delegates, update your delegates, and clear all of your delegates using the .NET and Java SDKs. Each segment will cover the same information. Go ahead and skip to section which applies to you. Full example code for this blog can be found in the Developer Community Code Share: Java, and .NET.
Java SDK
The first step is to retrieve all senders in your OneSpan Sign account. The code below shows you how to retrieve your signers, which comes as a page from OneSpan Sign, along with their emails and ids. The sender ids will be required in order to add/remove delegates. In the code below, the number of senders returned in the PageRequest is set to 5. The maximum number of senders that you can retrieve per page is 50. The first value in the PageRequest (variable ‘i’) is the starting point in the overall list of senders that should be returned. This variable is incremented as we step through our senders to keep track of where we are in the overall group of senders.
int i = 1; Map<String, Sender> accountMembers = client.getAccountService().getSenders(Direction.ASCENDING, new PageRequest(i,5)); while(!accountMembers.isEmpty()) { for (Map.Entry entry : accountMembers.entrySet()) { String email = (String) entry.getKey(); Sender sender = (Sender) entry.getValue(); System.out.println(email + ", " + sender.getId()); i++; } accountMembers = client.getAccountService().getSenders(Direction.ASCENDING, new PageRequest(i,5)); }
To add a delegate, you will need to retrieve your sender using your OneSpan Sign AccountService. A sender id, like the one retrieved in the last step, is required to retrieve a particular sender. Once you have your sender, you then build your DelegationUser object and call your OneSpan Sign client AccountService to add your delegate. Your user id is required to add delegates. Much like the senderId you used above to get your sender, the code above will get you that.
Sender user1 = client.getAccountService().getSender(sender1Id); DelegationUser delegationUser1 = DelegationUserBuilder.newDelegationUser(user1).build(); client.getAccountService().addDelegate(ownerId, delegationUser1);
To remove a delegate, you simply call on your OneSpan Sign client AccountService. Similar to adding delegates, you will need your user id and the sender id.
client.getAccountService().removeDelegate(ownerId, sender1Id);
You can also do a bulk update of your delegates list. Doing so will clear all of your current delegates and replace them with the ones defined in your list. To do this, all you need to do is create a list of delegate IDs and use the AccountService to update your delegates.
List<String> delegateIds = new ArrayList<String>(); delegateIds.add(sender1Id); delegateIds.add(sender2Id); delegateIds.add(sender3Id); client.getAccountService().updateDelegates(ownerId, delegateIds);
If you would like to know who is already a delegate on your account, you can access those, in list form with the following AccountService call.
List<DelegationUser> delegates = client.getAccountService().getDelegates(ownerId); int i = 1; for(DelegationUser delegate : delegates) { System.out.println("Delegate " + i + ": " + delegate.getName() + ", with email " + delegate.getEmail()); i++; }
Finally, if you wish to clear all of your delegates, the following line will accomplish that. Again, you will need your user id.
client.getAccountService().clearDelegates(ownerId);
.NET SDK
The first step is to retrieve all senders in your OneSpan Sign account. The code below shows you how to retrieve your signers, which comes as a page from OneSpan Sign, along with their emails and ids. The sender ids will be required in order to add/remove delegates. In the code below, the number of senders returned in the PageRequest is set to 5. The maximum number of senders that you can retrieve per page is 50. The first value in the PageRequest (variable ‘i’) is the starting point in the overall list of senders that should be returned. This variable is incremented as we step through our senders to keep track of where we are in the overall group of senders.
int i = 1; IDictionary<string, Sender> accountMembers = client.AccountService.GetSenders(Direction.ASCENDING, new PageRequest(i, 5)); while (accountMembers.Count != 0) { foreach (var s in accountMembers) { string email = s.Key.ToString(); string id = s.Value.Id; Debug.WriteLine(email + " " + id); i++; } accountMembers = client.AccountService.GetSenders(Direction.ASCENDING, new PageRequest(i, 5)); }
To add a delegate, you will need to retrieve your sender using your OneSpan Sign AccountService. A sender id, like the one retrieved in the last step, is required to retrieve a particular sender. Once you have your sender, you then build your DelegationUser object and call your OneSpan Sign client AccountService to add your delegate. Your user id is required to add delegates. Much like the senderId you used above to get your sender, the code above will get you that.
Sender user1 = client.AccountService.GetSender(sender1Id); DelegationUser delegationUser1 = DelegationUserBuilder.NewDelegationUser(user1).Build(); client.AccountService.AddDelegate(ownerId, delegationUser1);
To remove a delegate, you simply call on your OneSpan Sign client AccountService. Similar to adding delegates, you will need your user id and the sender id.
client.AccountService.RemoveDelegate(ownerId, sender1Id);
You can also do a bulk update of your delegates list. Doing so will clear all of your current delegates and replace them with the ones defined in your list. To do this, all you need to do is create a list of delegate IDs and use the AccountService to update your delegates.
List<string> delegateIds = new List<string>(); delegateIds.Add(sender1Id); delegateIds.Add(sender2Id); delegateIds.Add(sender3Id); client.AccountService.UpdateDelegates(ownerId, delegateIds);
If you would like to know who is already a delegate on your account, you can access those, in list form with the following AccountService call.
IList<DelegationUser> delegates = client.AccountService.GetDelegates(ownerId); int i = 1; foreach (var user in delegates){ Debug.WriteLine("Delegate " + i + ": " + user.Name + " with email " + user.Email); i++; }
Finally, if you wish to clear all of your delegates, the following line will accomplish that. Again, you will need your user id.
client.AccountService.ClearDelegates(ownerId);
Running Your Code
You can now go ahead and run your code. When retrieving your senders with the SDKs as shown above, I included the code that grabbed your senders’ email and id.
Furthermore, I also added the code that retrieved your current list of delegates, which prints out their full name and email.
Lastly, you will find below your list of users that can manage your transactions in your OneSpan Sign account.
There you go. You have successfully added delegates to your OneSpan Sign account. If you have questions regarding this blog or anything else concerning integrating OneSpan Sign into your application, visit the developer community forums: https://developer.onespan.com. That's it from me. Thank you for reading! If you found this post helpful, please share it on Facebook, Twitter, or LinkedIn.