OneSpan Sign for New Users: Check Package Status and Download Files with REST in Java
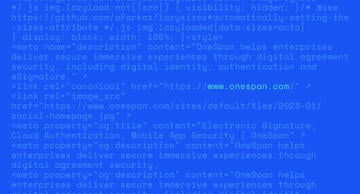
In this blog, I will follow up on the Create and Send a Package with REST in Java blog. I will show you some things you can do after sending the package, such as:
- Check the package/signing status
- Download the e-signed documents
- Download the evidence summary
Configuration
Initial Setup
Go ahead and create a new .java file for this tutorial. You can either start a new project or simply create a new class in a previously created project. I named my file "CheckStatusAndDownloadRest.java". Working with REST API requires the use of JSON. In order to work with it more easily, I will use an external library org.json. The jar file can be found here. If you require help on how to import external libraries, follow the steps in this previous blog.
Locating the Package ID of your Existing Project
For this example, you will need a packageId from a previously created document package. The two most likely methods to retrieve your packageId are to navigate to the package in the UI and retrieve it from the URL, or similarly in the previous blog, the packageId was returned in the HTTP response you received from you REST call.
The Code
We are now ready to write the code in your .java class. Copy the following code in your "CheckStatusAndDownloadRest.java" file. I will go over the code in greater detail below.
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; import java.io.FileOutputStream; import java.io.InputStream; import org.json.*; public class CheckStatusAndDownloadREST { public static void main(String[] args) throws MalformedURLException, IOException, JSONException { String API_KEY = "YOUR_API_KEY"; String url = "https://sandbox.esignlive.com/api/packages"; URL client = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/"); HttpURLConnection conn = (HttpURLConnection) client.openConnection(); conn.setRequestProperty("Content-Type", "application/json"); conn.setRequestProperty("Authorization", "Basic " + API_KEY); conn.setRequestProperty("Accept", "application/json"); int responseCode = ((HttpURLConnection) conn).getResponseCode(); if (responseCode == 200) { System.out.println(responseCode + " OK!"); BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream())); String inputLine; StringBuffer response = new StringBuffer(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); conn.disconnect(); JSONObject json = new JSONObject(response.toString()); System.out.println(json.getString("status")); } else { System.out.println("Request did not succeed."); } URL client2 = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/signingStatus"); HttpURLConnection conn2 = (HttpURLConnection) client2.openConnection(); conn2.setRequestProperty("Content-Type", "application/json"); conn2.setRequestProperty("Authorization", "Basic " + API_KEY); conn2.setRequestProperty("Accept", "application/json"); int responseCode2 = ((HttpURLConnection) conn2).getResponseCode(); if (responseCode2 == 200) { System.out.println(responseCode2 + " OK!"); BufferedReader in2 = new BufferedReader(new InputStreamReader(conn2.getInputStream())); String inputLine2; StringBuffer response2 = new StringBuffer(); while ((inputLine2 = in2.readLine()) != null) { response2.append(inputLine2); } in2.close(); conn2.disconnect(); JSONObject json2 = new JSONObject(response2.toString()); System.out.println(json2.getString("status")); if (json2.getString("status").equals("COMPLETED")) { URL client3 = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/documents/zip"); HttpURLConnection conn3 = (HttpURLConnection) client3.openConnection(); conn3.setRequestProperty("Content-Type", "application/json"); conn3.setRequestProperty("Authorization", "Basic " + API_KEY); conn3.setRequestProperty("Accept", "application/zip"); int responseCode3 = ((HttpURLConnection) conn3).getResponseCode(); System.out.println(responseCode3 + " OK!"); System.out.println("Downloading zip files...."); InputStream inputStream = conn3.getInputStream(); String saveFilePath = "C:/Users/your_account/Desktop/yourFiles.zip"; FileOutputStream outputStream = new FileOutputStream(saveFilePath); int bytesRead = -1; byte[] buffer = new byte[4096]; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } System.out.println("Zip files downloaded!"); outputStream.close(); inputStream.close(); conn3.disconnect(); URL client4 = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/evidence/summary"); HttpURLConnection conn4 = (HttpURLConnection) client4.openConnection(); conn4.setRequestProperty("Content-Type", "application/json"); conn4.setRequestProperty("Authorization", "Basic " + API_KEY); conn4.setRequestProperty("Accept", "application/pdf"); int responseCode4 = ((HttpURLConnection) conn4).getResponseCode(); System.out.println(responseCode4 + " OK!"); System.out.println("Downloading evidence summary...."); inputStream = conn4.getInputStream(); saveFilePath = "C:/Users/your_account/Desktop/evidenceSummary.pdf"; outputStream = new FileOutputStream(saveFilePath); bytesRead = -1; buffer = new byte[4096]; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } System.out.println("Evidence summary downloaded!"); outputStream.close(); inputStream.close(); conn4.disconnect(); } else { System.out.println("The package has not been signed yet!"); } } else { System.out.println("Request did not succeed!"); } } }
Let’s go over the code in closer detail. As before, the first two lines is where your connection info for your e-SignLive connection is defined. If you are on the production environment, change the url to https://apps.e-signlive.com/api. Make sure to replace the placeholder text with your API_KEY. In the Sandbox, your API_KEY can be found in the ACCOUNT page when you sign into your OneSpan Sign account. If in production, you will need to get this from support.
String API_KEY = "YOUR_API_KEY"; String url = "https://sandbox.esignlive.com/api/packages";
The next couple of lines is where you create your connection to make your GET request and set the appropriate header content-type, authorization, and accept values.
URL client = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/"); HttpURLConnection conn = (HttpURLConnection) client.openConnection(); conn.setRequestProperty("Content-Type", "application/json"); conn.setRequestProperty("Authorization", "Basic " + API_KEY); conn.setRequestProperty("Accept", "application/json");
Check the Status
In this section, you will need your package id that you have found previously. With it, you are ready to make your GET request to determine the status of your package. The two lines below is where we get the status code from the HTTP response message. If the request is successful, responseCode should print out 200.
int responseCode = ((HttpURLConnection) conn).getResponseCode();
If the request is successful, we create an input stream and read from the connection in a BufferedReader. The JSON content of the response is subsequently parsed into a JSONObject and printed out. The status of the package is given in the "status" property of the JSONObject.
System.out.println(responseCode + " OK!"); BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream())); String inputLine; StringBuffer response = new StringBuffer(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); JSONObject json = new JSONObject(response.toString()); System.out.println(json.getString("status"));
Retrieving the signing status of your package is done exactly the same way except for an addition of "/signingStatus" to the request url.
URL client2 = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/signingStatus"); HttpURLConnection conn2 = (HttpURLConnection) client2.openConnection(); conn2.setRequestProperty("Content-Type", "application/json"); conn2.setRequestProperty("Authorization", "Basic " + API_KEY); conn2.setRequestProperty("Accept", "application/json"); int responseCode2 = ((HttpURLConnection) conn2).getResponseCode(); if (responseCode2 == 200) { System.out.println(responseCode2 + " OK!"); BufferedReader in2 = new BufferedReader(new InputStreamReader(conn2.getInputStream())); String inputLine2; StringBuffer response2 = new StringBuffer(); while ((inputLine2 = in2.readLine()) != null) { response2.append(inputLine2); } in2.close(); conn2.disconnect(); JSONObject json2 = new JSONObject(response2.toString()); System.out.println(json2.getString("status"));
Download the Files
For the final part, I will show you how to download your package into a zip file on your desktop, as well as the evidence summary. The first couple of lines remain the same. As a reminder, make sure to add "/documents/zip" the request url.
if (json2.getString("status").equals("COMPLETED")){ URL client3 = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/documents/zip"); HttpURLConnection conn3 = (HttpURLConnection) client3.openConnection(); conn3.setRequestProperty("Content-Type", "application/json"); conn3.setRequestProperty("Authorization", "Basic " + API_KEY); conn3.setRequestProperty("Accept", "application/zip"); int responseCode3 = ((HttpURLConnection) conn3).getResponseCode(); System.out.println(responseCode3 + " OK!"); System.out.println("Downloading zip files....");
We then open the input stream from the HTTP connection. For this example, I’ve chosen to download the zip file on my desktop but you can specify any location you wish on your computer. In my example, do not forget to replace the placeholder text with your account name.
InputStream inputStream = conn3.getInputStream(); String saveFilePath = "C:/Users/your_account/Desktop/yourFiles.zip";
Next, open an output stream to write the zip file in the specified location. After all the data is written, go ahead and close the connection.
FileOutputStream outputStream = new FileOutputStream(saveFilePath); int bytesRead = -1; byte[] buffer = new byte[4096]; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } System.out.println("Zip files downloaded!"); outputStream.close(); inputStream.close(); conn3.disconnect();
To download the evidence summary, the code is almost identical to downloading the zip file, with the exception of the "Accept" header field. Make sure to change that to "application/pdf".
URL client4 = new URL(url + "/1edd9f11-9bcc-4587-94e8-aa6478bbda4d/evidence/summary"); HttpURLConnection conn4 = (HttpURLConnection) client4.openConnection(); conn4.setRequestProperty("Content-Type", "application/json"); conn4.setRequestProperty("Authorization", "Basic " + API_KEY); conn4.setRequestProperty("Accept", "application/pdf"); int responseCode4 = ((HttpURLConnection) conn4).getResponseCode(); System.out.println(responseCode4 + " OK!"); System.out.println("Downloading evidence summary...."); inputStream = conn4.getInputStream(); saveFilePath = "C:/Users/your_account/Desktop/evidenceSummary.pdf"; outputStream = new FileOutputStream(saveFilePath); bytesRead = -1; buffer = new byte[4096]; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } System.out.println("Evidence summary downloaded!"); outputStream.close(); inputStream.close(); conn4.disconnect();
Running the code
With your class completed, it is time to run the Java file. Press the run button in your IDE. The package I checked was already signed, so the output looks like the following:
If you browse to the location you specified to save your zip file and evidence summary, you will find your files (if your package was completed):
There you go. You have successfully checked the status of your package, downloaded the files from your package and the evidence summary.
If you have questions regarding this blog or anything else concerning integrating OneSpan Sign into your application, visit the developer community forums:
https://developer.esignlive.com. That's it from me. Thank you for reading! If you found this post helpful, please share it on Facebook, Twitter, or LinkedIn.
Haris Haidary
Junior Technical Product Evangelist
LinkedIn | Twitter
OneSpan Sign for New Users Blog Series