OneSpan Sign How To: Create and Send A Package with REST in PHP
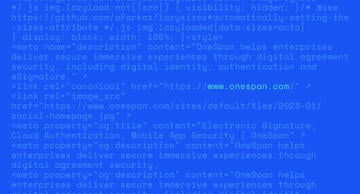
In a previous blog, I showed you how to create a simple PHP web form application with OneSpan Sign. However, in the code I broke down the process into three separate requests. Namely, after the user has filled-out the form, three HTTP calls are made to OneSpan Sign:
- Create Package
- Upload Document
- Send Package
In this blog, I will show you how you can simplify the process and make above requests in one single call.
The Code
If you don’t already have PHP installed, you can download it from the official website. The complete example code is also available on the Developer Community Code Share.
Let's get started. In your favorite text editor, create a new file named "CreateAndSendPackage.php" and save it in a location of your choice. You can go ahead and copy the code below. I will go over it in more detail further down.
<?php define("API_URL", "https://sandbox.esignlive.com/api/packages"); define("MULTIPART_BOUNDARY", "----WebKitFormBoundary7MA4YWxkTrZu0gW"); $api_headers = array( "Authorization: Basic api_key", "Accept: application/json; esl-api-version=11.0", "Content-Type: multipart/form-data; boundary=\"" . MULTIPART_BOUNDARY . "\"", ); $json = array( 'type' => 'PACKAGE', 'status' => 'SENT', 'roles' => array( array( 'id' => 'Signer1', 'type' => 'SIGNER', 'signers' => array( array( 'email' => '[email protected]', 'firstName' => 'John', 'lastName' => 'Smith', 'id' => 'Signer1', ) ) , ) , array( 'id' => 'Sender1', 'type' => 'SIGNER', 'signers' => array( array( 'email' => '[email protected]', 'firstName' => 'Mike', 'lastName' => 'Smith', 'id' => 'Sender1', ) ) , ) , ) , 'name' => 'PHP Application Example', 'documents' => array( array( 'name' => 'Sample Contract', 'id' => 'contract', 'extract' => 'true' ) ) ); $postdata = "--" . MULTIPART_BOUNDARY . "\r\n"; $postdata .= "Content-Disposition: form-data; name=\"file\"; filename=\"sample_contract2.pdf\"\r\n\r\n"; $postdata .= file_get_contents("documents/sample_contract2.pdf"); $postdata .= "\r\n\r\n"; $postdata .= "--" . MULTIPART_BOUNDARY . "\r\n"; $postdata .= "Content-Disposition: form-data; name=\"payload\"\r\n\r\n"; $postdata .= json_encode($json); $postdata .= "\r\n\r\n"; $postdata .= "--" . MULTIPART_BOUNDARY . "--\r\n"; $curl = curl_init(API_URL); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); curl_setopt($curl, CURLOPT_POST , true); curl_setopt($curl, CURLOPT_POSTFIELDS , $postdata); curl_setopt($curl, CURLOPT_HTTPHEADER , $api_headers); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_exec($curl); $response = curl_multi_getcontent($curl); curl_close($curl); echo mb_convert_encoding(print_r(json_decode($response, true), true), 'CP932', 'UTF-8'); ?>
Now, let’s go over the code in greater detail. The first couple of lines defines the constant needed in order to make RESTful calls to OneSpan Sign's API. The API_URL constant is the endpoint URL where we will make our request to. The boundary is an arbitrary value and separates the multiple "parts" of a multipart/form-data request.
define("API_URL", "https://sandbox.esignlive.com/api/packages"); define("MULTIPART_BOUNDARY", "----WebKitFormBoundary7MA4YWxkTrZu0gW");
Next, we define the HTTP headers required to make a POST request to OneSpan Sign. Here, make sure to replace the api_key placeholder with your own value.
$api_headers = array( "Authorization: Basic api_key", "Accept: application/json; esl-api-version=11.0", "Content-Type: multipart/form-data; boundary=\"" . MULTIPART_BOUNDARY . "\"", );
Then, we build the json payload that will be included along with the PDF document in the POST request to OneSpan Sign.
$json = array( 'type' => 'PACKAGE', 'status' => 'SENT', 'roles' => array( array( 'id' => 'Signer1', 'type' => 'SIGNER', 'signers' => array( array( 'email' => '[email protected]', 'firstName' => 'John', 'lastName' => 'Smith', 'id' => 'Signer1', ) ) , ) , array( 'id' => 'Sender1', 'type' => 'SIGNER', 'signers' => array( array( 'email' => '[email protected]', 'firstName' => 'Mike', 'lastName' => 'Smith', 'id' => 'Sender1', ) ) , ) , ) , 'name' => 'PHP Application Example', 'documents' => array( array( 'name' => 'Sample Contract', 'id' => 'contract' ) ) );
Once you've defined the json payload, the next step is to build the multipart/form-data request, as shown below.
$postdata = "--" . MULTIPART_BOUNDARY . "\r\n"; $postdata .= "Content-Disposition: form-data; name=\"file\"; filename=\"sample_contract2.pdf\"\r\n\r\n"; $postdata .= file_get_contents("documents/sample_contract2.pdf"); $postdata .= "\r\n\r\n"; $postdata .= "--" . MULTIPART_BOUNDARY . "\r\n"; $postdata .= "Content-Disposition: form-data; name=\"payload\"\r\n\r\n"; $postdata .= json_encode($json); $postdata .= "\r\n\r\n"; $postdata .= "--" . MULTIPART_BOUNDARY . "--\r\n";
Finally, you execute your cURL request and echo the response sent by OneSpan Sign.
$curl = curl_init(API_URL); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); curl_setopt($curl, CURLOPT_POST , true); curl_setopt($curl, CURLOPT_POSTFIELDS , $postdata); curl_setopt($curl, CURLOPT_HTTPHEADER , $api_headers); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_exec($curl); $response = curl_multi_getcontent($curl); curl_close($curl); echo mb_convert_encoding(print_r(json_decode($response, true), true), 'CP932', 'UTF-8');
Running Your Code
Open your command prompt and change the current directory to the location where you saved your "CreateAndSendPackage.php" file. Then, enter the following lines:
php CreateAndSendPackage.php
OneSpan Sign will then return a package id as a response, which will be printed to the command prompt window.
If you have questions regarding this blog or anything else concerning integrating OneSpan Sign into your application, visit the developer community forums: https://developer.OneSpan.com/. That's it from me. Thank you for reading! If you found this post helpful, please share it on Facebook, Twitter, or LinkedIn.
Haris Haidary
Junior Technical Evangelist
LinkedIn | Twitter