Orchestration SDK – PIN-Change Integration
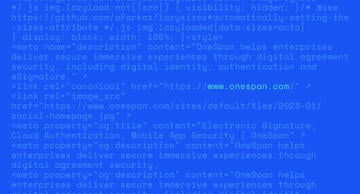
When integrating the Orchestration SDK to your mobile application, you sign-up for the numerous features that enhance the security of your application. Allowing your end-user to change their PIN securely on their mobile app is one of these features. In today’s blog, we will explore the Change PIN activity with a sample code empowered by OneSpan’s Orchestration SDK and the different possible flows when implementing the ChangePINCallback
.
Prerequisites:
- Android 4.1 or later.
- A registered user on the sandbox environment of the OneSpan Intelligent Adaptive Authentication. Here’s a full reference on how to do so.
- The MSS Orchestration SDK’s sample application.
Note: the PIN
could be referred to as Password
in the sample application. Through this tutorial, our sample code will only use the PIN terminology.
Note: all commands are exchanged and interpreted explicitly between the TID platform and the client application server through the orchestration-commands/
endpoint.
Changing the PIN Process
The end-user triggers the process to change the old PIN on their trusted device from their mobile application, which integrates the Orchestration SDK. The mobile application will be required to implement the ChangePINCallback
and override its methods as we will discuss in details below.
Orchestrator builder and Client Device Data Collector (CDDC)
The OrchestrationCallback
object will be created with the current Context, which will be passed to the OrchestrationCallback
as an argument, referenced with the this
keyword. Afterwards, an object of the inner Builder class of the Orchestrator
class will be created. The purpose of the Builder object is to create an Orchestrator
object following the concept of the Builder design pattern, as seen below.
// Orchestration
private Orchestrator orchestrator;
private String userIdentifier;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_change_PIN);
Toolbar toolbar = findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
// Get orchestrator instance
orchestrationCallback = new SampleOrchestrationCallback(this);
Orchestrator.Builder builder = new Orchestrator.Builder();
orchestrator = builder
.setDigipassSalt(Constants.SALT_DIGIPASS)
.setStorageSalt(Constants.SALT_STORAGE)
.setContext(getApplicationContext())
.setDefaultDomain(Constants.DOMAIN)
.setCDDCParams(CDDCUtils.getCDDCParams())
.setErrorCallback(orchestrationCallback)
.setWarningCallback(orchestrationCallback)
.build();
// Set values for the CDDC when available
CDDCUtils.configure(orchestrator.getCDDCDataFeeder());
Before invoking the build
method to complete the creation of the Orchestrator
object, the following arguments should be defined as shown in the code above:
Hardcoded
salts, which will be used for diversification with certain security features (e.g. device binding, secure storage). These salts will be derived by the Orchestration SDK Client to complicate reverse-engineering attacks.- Default domain, required if the Customer Application Server integrates OneSpan Authentication Server. If the Customer Mobile Application must manage multiple domains, the default domain can be dynamically overwritten for each action (e.g.
startActivation
. - A
CDDCParams
object, which will define the parameters for device data collection. These parameters are optional. - An
OrchestrationErrorCallback
object, in case of errors, will be used to throw them from the Orchestration SDK Client to the Customer Mobile Application. - An
OrchestrationWarningCallback
object, in case of warnings, will be used to throw them from the Orchestration SDK Client to the Customer Mobile Application. - After the
Orchestrator
object is built successfully, the static configure method of theCDDCUtilsclass
will be called to set up the values for the CDDC data from theorchestrator.getCDDCDataFeeder()
Changing PIN parameters
In the process of changing the PIN, the parameters will be set in onStartChangePIN()
method. This method takes a View object as an argument to display a message to the end-user telling them that their request is in progress. Afterward, the orchestrator
object will set the authentication method required to changes the PIN, and the keypad will be used for capturing the PIN. This keypad could be the default provided in the Orcherstration SDK or a custom keypad developed by the customer.
In the next step, ChangePINParams
object will be initialized. The OrchestrationUser
and the current ChangePINActivity
will be provided as parameters along with the index of the cryptographic application, which is used to sign the authentication request. After that, the Orchestrator
will call the startChangePIN()
method passing all the necessary parameters to change the PIN.
public void onStartChangePIN(View view) {
// Display progress dialog and start PIN changing
progressDialog = UIUtils.displayProgress(this, getString(R.string.dialog_progress_change_pwd));
orchestrationCallback.setProgressDialog(progressDialog);
// Used for custom PIN instead of default one
orchestrator.setUserAuthenticationCallback(orchestrationCallback, new UserAuthenticationCallback.UserAuthentication[]{UserAuthenticationCallback.UserAuthentication.PIN});
// Initializing params
ChangePINParams PINParams = new ChangePINParams();
PINParams.setOrchestrationUser(new OrchestrationUser(userIdentifier));
PINParams.setChangePINCallback(ChangePINActivity.this);
PINParams.setCryptoAppIndex(CryptoAppIndex.SECOND);
orchestrator.startChangePIN(PINParams);
}
To be ready for all possible scenarios, the Orchestration SDK requires the client application to override all the ChangePINCallback
methods. It starts with the onChangePINStepComplete
which has the CommandSender
object to communicate with the TID platform, and it receives an orchestration command as a parameter which will be sent later to the server.
In the CommandSender
object, there are two callback methods to be defined in this step. The onCommandSendingSuccess()
which will be run to execute the upcoming server command on the phone in case of a successful reception. In addition, there is the onCommandSendingFailure()
which will be executed in case of any failure. Below is the sample code for onChangePINStepComplete
method.
@Override
public void onChangePINStepComplete(String command) {
CommandSender commandSender = new CommandSender(getApplicationContext(), new CommandSenderCallback() {
@Override
public void onCommandSendingSuccess(String serverCommand) {
orchestrator.execute(serverCommand);
}
@Override
public void onCommandSendingFailure() {
// Hide progress dialog and display error message
UIUtils.hideProgress(progressDialog);
UIUtils.displayAlert(ChangePINActivity.this, getString(R.string.dialog_error_title), getString(R.string.dialog_error_content_sending));
}
});
commandSender.execute(command);
}
The rest of the methods of the ChangePINCallback
are below with their description:
onChangePINSuccess
: it will be called after a successful PIN reset and display to the end-user the message provided toUIUtils.displayAlert()
method.onChangePINAborted
: it will be called if the PIN change has been aborted and will convey the message to the end-user.onChangePINInputError
: it will be called if an error occurs during the change PIN process due to incorrect user input. The possible errors are listed in theOrchestrationErrorCodes
class.
@Override
public void onChangePINSuccess() {
UIUtils.hideProgress(progressDialog);
UIUtils.displayAlert(this, getString(R.string.dialog_title_change_PIN), getString(R.string.dialog_content_change_PIN_success));
}
@Override
public void onChangePINAborted() {
UIUtils.hideProgress(progressDialog);
UIUtils.displayAlert(this, getString(R.string.dialog_title_change_PIN), getString(R.string.dialog_content_change_PIN_abortion));
}
@Override
public void onChangePINInputError(PINError error) {
UIUtils.hideProgress(progressDialog);
UIUtils.displayAlert(this, getString(R.string.dialog_error_title), ErrorUtils.getErrorMessage(this, error.getErrorCode()));
}
This concludes the tutorial on how to utilize the Orchestration SKD to integrate the PIN change feature to your mobile application. If you have any questions, reach out to us on the OneSpan Forums.
Orherstration SDK related articles:
- Orchestration SDK: Authenticator Activation on Android Studio
- Understanding Callback Methods with Examples