OneSpan Sign How To: Creating a Callback Event Notification Listener in PHP
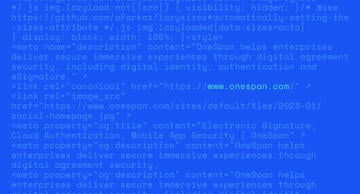
In a previous blog, I showed you how to create a simple callback event notification listener in C# and listen for the "PACKAGE_COMPLETE" event in order to download signed documents. In this blog, I will show you how to do the same in PHP. Let's get straight into it.
Requirements
Register for Event Notifications
Before the OneSpan Sign system can notify you of an event, you must first register to be notified in case of an event. You can follow this feature guide on how to register for event notifications.
SublimeText
For this blog, I will use the SublimeText text editor. If you do not already have it, you can download it for free, here.
Ngrok
In order to receive callback event notifications, you will need a publicly accessible URL to hit and work. Your localhost server on its own will not work. Ngrok is a very simple and easy to use tool that creates a secure tunnel on your local machine along with a public URL you can use for browsing your local site. This saves you the trouble of deploying your web application.
XAMPP
I’ll also be using XAMPP, which is a free open-source cross-platform web server with PHP and PERL interpreters. It’s simple, lightweight and allows you to test your scripts locally. You can download it for free from their official website.
The Code
You can download the example code from the Developer Community Code Share. Go ahead and create a new file in SublimeText named "callback.php". Copy the code below. I will go over it shortly.
<?php $data = file_get_contents('php://input'); $json = json_decode($data, true); $packageId = print_r($json['packageId'], true); $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => "https://sandbox.esignlive.com/api/packages/".$packageId."/documents/zip", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_SSL_VERIFYHOST => false, CURLOPT_SSL_VERIFYPEER => false, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "GET", CURLOPT_HTTPHEADER => array( "authorization: Basic api_key" ), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { file_put_contents("C:/Users/hhaidary/Desktop/documents_".$packageId.".zip", $response); }
The php://input wrapper is a read-only stream that allows you to read raw data from the request body. In the case of POST requests, it is preferable to use php://input instead of $HTTP_RAW_POST_DATA as it does not depend on special php.ini directives.
$data = file_get_contents('php://input');
Next, we json_decode the JSON encoded string sent from OneSpan Sign and convert it into an array.
$json = json_decode($data, true);
In my example, I am only interested in the package id since this is the only information I need to download signed documents.
$packageId = print_r($json['packageId'], true);
Then, it's a simple matter of using the cURL library in order to send your HTTP request to OneSpan Sign's API and download the signed documents.
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => "https://sandbox.esignlive.com/api/packages/".$packageId."/documents/zip", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_SSL_VERIFYHOST => false, CURLOPT_SSL_VERIFYPEER => false, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "GET", CURLOPT_HTTPHEADER => array( "authorization: Basic api_key"), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { file_put_contents("C:/Users/hhaidary/Desktop/documents_".$packageId.".zip", $response); }
Running Your Code
Go ahead and run your local server with XAMPP. Then, open a command prompt and change the directory to the location where you saved your ngrok executable. Enter the following command:
ngrok http [port] -host-header="localhost:[port]"
Below is a screenshot of what you can expect after running the command:
Then, login to your OneSpan Sign account and browse to the Admin page. Enter in the "Callback URL" field the URL to your callback listener (i.e. http://f3e93555.ngrok.io/callback.php) and register for the "transaction complete" event. Finally, create, send, and complete a test package. You should be able to view the signed documents as a zipped file in the location where you chose to save it.
If you have questions regarding this blog or anything else concerning integrating OneSpan Sign into your application, visit the developer community forums: https://developer.OneSpanSign.com/. That's it from me. Thank you for reading! If you found this post helpful, please share it on Facebook, Twitter, or LinkedIn.
Haris Haidary
Junior Technical Evangelist