OneSpan Sign Developer: Callback Event Notification – PHP
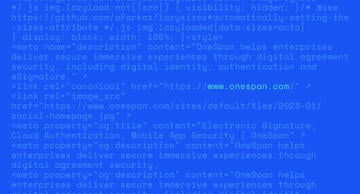
Once your integration is configured to send transactions to recipients via email or by using embedded signing, the next logical step is to enable your integration to automate post e-signature tasks. Setting callback listeners and leveraging event notifications is strongly recommended.
In this blog, we will demonstrate a working example in PHP language, showcase how to setup a web application, and respond to OneSpan Sign callback notifications. Without further delay, let’s get started!
Callback Event Notification in Action
Before beginning a PHP project, be sure you complete the following:
- Register for Event Notifications: Before the OneSpan Sign system can notify you of an event, you must turn on notifications. Follow this feature guide to learn how to register. In our example, we will simply register from the web portal.
- Downloading XAMPP: XAMPP is a free open-source cross-platform web server with PHP and PERL interpreters. It’s simple, lightweight, and allows you to test your scripts locally.
- Download Ngrok
The Code
Download the complete source code. Feel free to copy the necessary files directly to your existing project.
The main entrance of the project is the callback.php file, where we will only handle POST requests:
if ($_SERVER["REQUEST_METHOD"] == "POST") { …… }
Once a transaction get completed, OneSpan Sign will make a POST request to the registered URL with the following example JSON payload:
{ "@class": "com.silanis.esl.packages.event.ESLProcessEvent", "name": "PACKAGE_COMPLETE", "sessionUser": "0787be84-f095-44c7-ba00-787093df86fc", "packageId": "KHiRfOXgKK0gpVWwwpFOSNy6o34=", "message": null, "documentId": null }
The corresponding code to unpack this request looks like this:
$headerArray = apache_request_headers(); if (array_key_exists('Authorization', $headerArray)) { $callbackKey = $headerArray['Authorization']; } $data = file_get_contents('php://input'); $payload = json_decode($data, true); $packageId = $payload['packageId']; $eventNmae = $payload['name'];
After we have successfully received the callback request, don’t forget to return an HTTP status code 200 and close the connection:
// buffer all upcoming output ob_start(); http_response_code(200); // get the size of the output $size = ob_get_length(); // send headers to tell the browser to close the connection header("Content-Length:".$size); header('Connection: close'); // flush all output ob_end_flush(); ob_flush(); flush();
As our main business logic, we will simply download the signed documents if the event name equals “PACKAGE_COMPLETE”, based on the data passed by the callback request:
if ($eventNmae == 'PACKAGE_COMPLETE') { $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => $apiurl."/api/packages/". $packageId. "/documents/zip", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_SSL_VERIFYHOST => false, CURLOPT_SSL_VERIFYPEER => false, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "GET", CURLOPT_HTTPHEADER => array( "authorization: Basic ".$apikey, ), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { file_put_contents($filepath."/documents_" . $packageId . "_" . date("Y_m_d_H_i_s") . ".zip", $response); } }
Perform a Test
Go ahead and run your local server with XAMPP. Then, open a command prompt and change the directory to the location where you saved your ngrok executable. Enter the following command:
ngrok http [port] -host-header="localhost:[port]"
Next, login to your OneSpan Sign account, browse to the Admin page, and modify the domain of your callback URL.
Finally, create, send, and complete a test package. You should be able to view the signed documents as a zipped file in the location of your choice. The moment OneSpan Sign sends a callback notification to your listener, you’ll see the message below at your output console.
If you have any questions regarding this blog or anything else concerning integrating OneSpan Sign into your application, visit the Developer Community Forums. Your feedback matters to us!