OneSpan Sign Developer: SMS Authentication Plugin
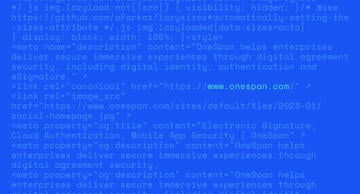
Different countries around the world have different formats for their phone numbers. For example, phone numbers in Poland are nine digits long, while there is no set length for phone numbers in Italy. If you are developing your own front end page and your e-signature workflow includes SMS Authentication, you need to include an HTML component to account for different standards in international telephone numbers. In this blog, we will demonstrate one “intl-tel-input” plugin that allows you to easily configure your OneSpan Sign deployment to include international phone numbers.
Below, we’ve included a GIF of the plugin in action:
Note in the demonstration these three key features:
- The plugin includes a dropdown menu with a country list and codes. This list can be customized for your target areas.
- When integrated with a second plugin called “Formatphonenumber,” your input will automatically be formatted to the phone number for the country selected.
- The plugin includes a built-in number validator, or you can easily add a customized validator yourself.
You can download the plugin code here.
Programming the SMS Authentication Plugin
In the HTML page, you first need to introduce the dependencies as described below:
<!-- jQuery --> <script src="js/jquery/jquery.min.js"></script> <!-- intl-tel-input --> <script src="js/intl-tel-input/js/intlTelInput.min.js"></script> <!-- formatphonenumber --> <script src="js/formatphonenumber/formatphonenumber.js"></script>
In this demo, we used two open source plugins:
- Intl-tel-input: A JavaScript plugin for entering and validating international telephone numbers. We used the jQuery-dependent version in the code. If necessary, they now also support a pure JavaScript version in their latest release.
- Formatphonenumber: Formats phone numbers to align with a selected country’s style, also depends on jQuery.
From there, we can code our page. The intl-tel-input component should be an “input” tag with id of “smsPhone”, which should be followed.
<div id="sms"> <label for="smsPhone">Phone Number: </label> <input type="tel" id="smsPhone"> <button id="submit">Submit</button> </div>
In our customized JavaScript code, we can initial the intl-tel-input plugin in this way:
//init intlTelInput $("#smsPhone").intlTelInput({ onlyCountries: ['ca', 'us', 'au'], preferredCountries:[], autoHideDialCode: false, utilsScript: "js/intl-tel-input/js/utils.js" });
Note:
- The “onlyCountries” attribute defines the country list.
- Change “utilsScript” path according to your project structure.
- Refer to their website for other available features.
Each time a user selects a country from the list, the intl-tel-input plugin will add a “placeholder” attribute to the HTML element, which can be used to format number:
var updateDataFormat = function(){ var dataFormat = $('#smsPhone').attr("placeholder").replace(/\d/g, "#"); $('#smsPhone').formatPhoneNumber({ format: dataFormat }); // console.log(dataFormat); }
In addition, this format function will need to be bound to HTML events:
//once the country is changed $("#smsPhone").on("countrychange", function(e, countryData) { $("#smsPhone").val(""); updateDataFormat(); }); //once the input is on focus $("#smsPhone").on("focus", function(e, countryData) { updateDataFormat(); });
When submitting, we separately retrieve country codes and phone numbers and print them in the console. This is where you can add your phone number validation before sending a package:
$("#submit").on("click", function() { var phoneNumber = $("#smsPhone").val().replace(/\D/g, "").replace(/^0+/, ""); var countryCode = $("#smsPhone").intlTelInput("getSelectedCountryData").dialCode; console.log(countryCode + phoneNumber); });
Finally, before launching, please always make sure the phone number format is consistent with the number used by OneSpan Sign. Go to your web portal to create a test package from your dashboard with the signer’s SMS Authentication selected. Then you can retrieve the package’s metadata and compare the format OneSpan Sign is using to yours.
We have successfully built an international phone number input component. Feel free to modify the code as you see fit.
Guides related to SMS Authentication
- If you’re just getting started with OneSpan Sign, our Quick Start Guides will walk you through how to create and send your package using APIs or SDKs.
- To add additional security, such as SMS verifying the identity of the signer, refer to our Signer Authentication Guide.
- If you were not familiar with adding a signer to a package, or you want to know more about other operations, refer to the Signer Management Guide.
If you have any questions regarding this blog or anything else concerning integrating OneSpan Sign into your application, visit the Developer Community Forums. Your feedback matters to us!